Introduction to Creating Image Tags in HTML using JavaScript
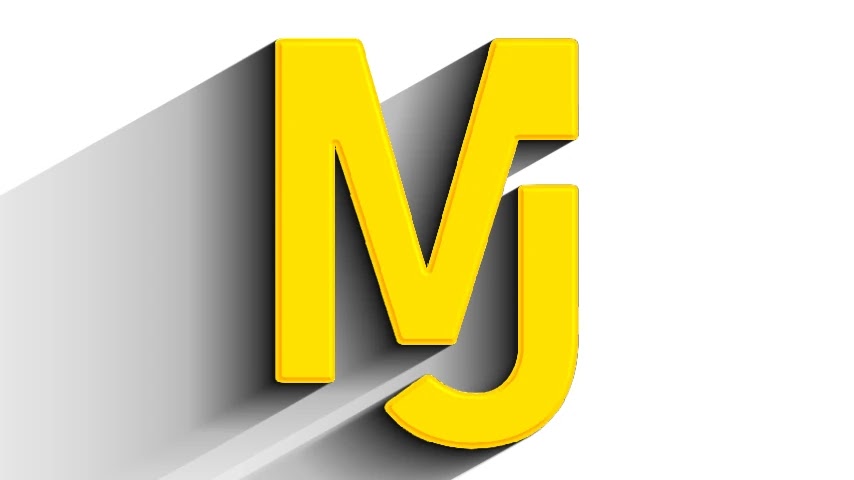
Title:
Objective:
This tutorial aims to teach you the basics of programming by demonstrating how to create image HTML tags using JavaScript. You will learn how to display images on a webpage using the <img>
tag, and manipulate their properties using JavaScript.
Prerequisites: Before starting this tutorial, it is assumed that you have a basic understanding of HTML, CSS, and JavaScript.
Note: To run the JavaScript code snippets, you need a web browser with a developer console.
Let's get started!
Easy:
Snippet 1: Creating Image Tag without JavaScript
Step 1: Open a new HTML file in your favorite code editor.
Step 2: Add the following code inside the <body>
tag:
<img src="image.jpg" alt="Description of the image">
In the code snippet above, we use the <img>
tag to create an image tag. The src
attribute specifies the URL or path to the image you want to display. The alt
attribute provides alternative text that will be displayed if the image fails to load.
Step 3: Save the HTML file with the desired name and extension (e.g., index.html
).
Output: The image specified in the src
attribute will be displayed on the webpage with the given alternative text.
Medium:
Snippet 2: Dynamically Changing Image Source with JavaScript
Step 1: Start with the HTML structure similar to Snippet 1.
Step 2: Add an id
attribute to the <img>
tag. For example:
<img id="myImage" src="placeholder.jpg" alt="Description of the image">
Step 3: Add the following JavaScript code just before the closing </body>
tag:
<script> var img = document.getElementById("myImage"); // Changes the source of the image to a new URL img.src = "new_image.jpg"; </script>
In this code snippet, we retrieve the image tag using its id
from the DOM (Document Object Model) using document.getElementById()
. Then, we manipulate the src
attribute of the image tag using JavaScript to change the image source dynamically.
Step 4: Save the HTML file and open it in a web browser.
Output: Initially, the image displayed will be the one specified in the src
attribute. However, when the JavaScript code is executed, the src
attribute value will change to the new image source specified in the code. The web browser will dynamically update the displayed image accordingly.
Hard:
Snippet 3: Changing Image Source on Button Click
Step 1: Prepare the HTML structure as in Snippet 2.
Step 2: Add the following JavaScript code just before the closing </body>
tag:
<script> var img = document.getElementById("myImage"); function changeImage() { if (img.src.endsWith("new_image.jpg")) { img.src = "image.jpg"; } else { img.src = "new_image.jpg"; } } </script>
In this code snippet, we define a JavaScript function called changeImage()
. Inside the function, we check the current src
value of the image. If it ends with "new_image.jpg", we change the src
attribute to "image.jpg", and vice versa.
Step 3: Add the following button code after the <img>
tag:
<button onclick="changeImage()">Change Image</button>
This code creates a button with the label "Change Image" and attaches an onclick
event handler that calls the changeImage()
function when the button is clicked.
Step 4: Save the HTML file and open it in a web browser.
Output: Initially, the image displayed will be the one specified in the src
attribute. When you click the "Change Image" button, the image source will toggle between "image.jpg" and "new_image.jpg". This action will dynamically update the displayed image each time the button is clicked.
Congratulations! You have learned the basics of creating image HTML tags using JavaScript. These code snippets will help you understand the fundamental concepts required to manipulate image tags and their properties. Feel free to experiment and modify the code to strengthen your understanding. With this knowledge, you can further explore other programming concepts in web development. Happy coding!
© Manajmnt code
Comments
Post a Comment
message